Models¶
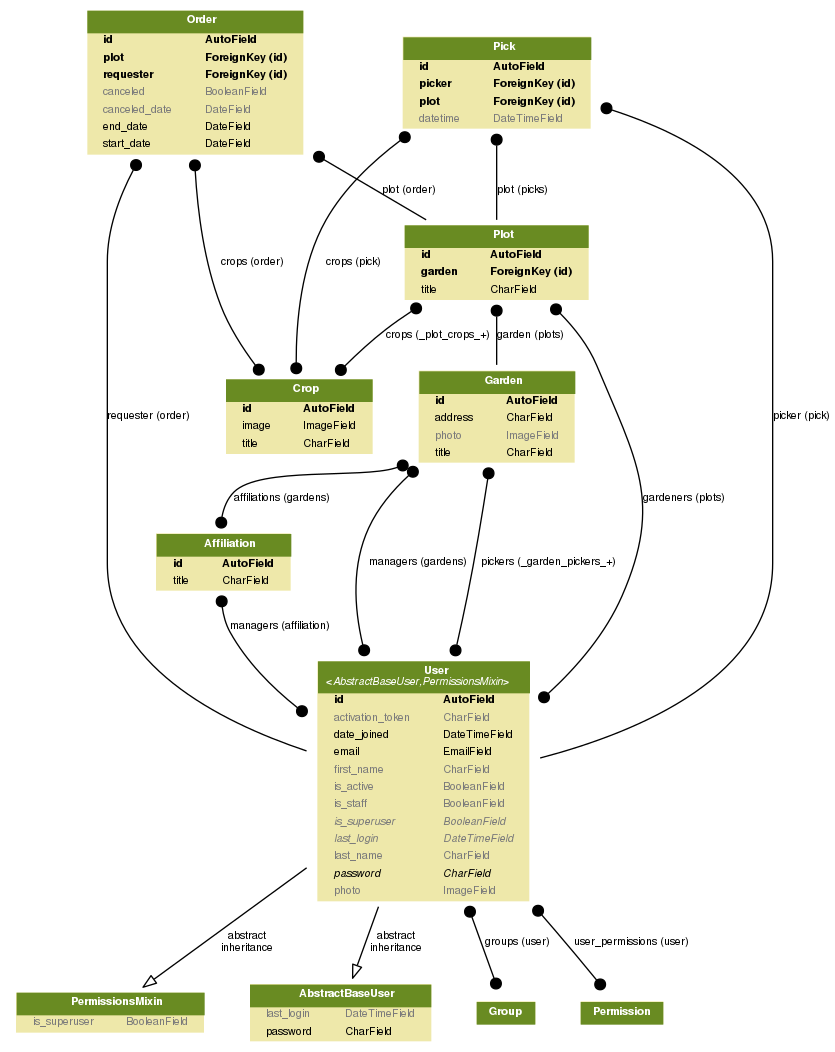
Querying objects¶
-
class
gardenhub.managers.
OrderQuerySet
(model=None, query=None, using=None, hints=None)¶ Custom QuerySet for advanced filtering of orders.
The following fields enable filtering orders via
Order.objects
. For example,from gardenhub.models import Order # All open orders orders = Order.objects.open() # All open orders that haven't been picked today orders = Order.objects.open().unpicked_today()
-
active
()¶ Orders that are happening right now.
-
closed
()¶ Orders that have finished or were canceled.
-
inactive
()¶ All orders that aren’t happening right now.
-
open
()¶ Orders that have not finished but also may not have begun.
-
picked_today
()¶ Orders that have at least one Pick from today.
-
unpicked_today
()¶ Orders that have no Picks from today.
-
upcoming
()¶ Orders that have not yet begun but are scheduled to happen.
-
Full model reference¶
-
class
gardenhub.models.
Affiliation
(*args, **kwargs)¶ A group of affiliated gardens.
-
exception
DoesNotExist
¶
-
exception
MultipleObjectsReturned
¶
-
exception
-
class
gardenhub.models.
Crop
(*args, **kwargs)¶ A crop represents an item that may be picked, such as a zuchini or an orange. Crops are stored in a master list, managed by the site admin, and may be listed on Orders or Picks.
-
exception
DoesNotExist
¶
-
exception
MultipleObjectsReturned
¶
-
exception
-
class
gardenhub.models.
Garden
(*args, **kwargs)¶ A whole landscape, divided into many plots. Managed by Garden Managers.
-
exception
DoesNotExist
¶
-
exception
MultipleObjectsReturned
¶
-
exception
-
class
gardenhub.models.
Order
(*args, **kwargs)¶ A request from a Gardener or Garden Manager to enlist a particular Plot for picking over a specified number of days.
-
exception
DoesNotExist
¶
-
exception
MultipleObjectsReturned
¶
-
get_picks
()¶ Return the list of Picks that occurred on this Order’s plot within this Order’s timeframe.
-
get_status_icon
()¶ Returns a Semantic UI status icon class string depending on this order’s status. This may be removed in the future.
-
is_active
()¶ Whether this Order is active.
-
is_closed
()¶ Whether this Order is closed.
-
is_open
()¶ Whether this Order is open.
-
progress
()¶ Percentage this order is complete, as a decimal between 0-100.
-
was_picked_today
()¶ True if at least one Pick was submitted today for the Order’s Plot.
-
exception
-
class
gardenhub.models.
Pick
(*args, **kwargs)¶ A submission by a picker signifying that certain Crops have been picked from a particular Plot at a particular time.
-
exception
DoesNotExist
¶
-
exception
MultipleObjectsReturned
¶
-
inquirers
()¶ People to notify about this pick.
-
exception
-
class
gardenhub.models.
Plot
(*args, **kwargs)¶ Subdivision of a Garden, allocated to a Gardener for growing food.
-
exception
DoesNotExist
¶
-
exception
MultipleObjectsReturned
¶
-
exception
-
class
gardenhub.models.
User
(*args, **kwargs)¶ Custom user class for GardenHub users. This is necessary because we want to authorize users by their email address (and provide a few extra fields).
-
exception
DoesNotExist
¶
-
exception
MultipleObjectsReturned
¶
-
can_edit_garden
(garden)¶ Can the given user manage this garden? True if the user is listed in Garden.managers for that garden. False otherwise.
-
can_edit_order
(order)¶ Can the given user manage this order? True if the user can edit Order.plot for that order. False otherwise.
-
can_edit_plot
(plot)¶ Can the given user manage this plot? True if the user is listed in Plot.gardeners for that plot, OR the user is listed in Garden.managers for the garden in Plot.garden. False otherwise.
-
clean
()¶ Hook for doing any extra model-wide validation after clean() has been called on every field by self.clean_fields. Any ValidationError raised by this method will not be associated with a particular field; it will have a special-case association with the field defined by NON_FIELD_ERRORS.
-
email_user
(subject, message, from_email=None, **kwargs)¶ Send an email to this user.
-
get_full_name
()¶ Return the first_name plus the last_name, with a space in between.
-
get_gardens
()¶ Return all the Gardens the given user can edit.
-
get_orders
()¶ Return all Orders for the given user’s Plots and Gardens.
-
get_peers
()¶ Return all the Users within every Plot and Garden that you manage, except yourself.
-
get_picker_gardens
()¶ Return all Gardens where the user is in Garden.picker.
-
get_picker_orders
()¶ Return all Orders this user is assigned to fulfill.
-
get_plots
()¶ Return all the Plots the given user can edit. Users can edit any plot which they are a gardener on, and any plot in a garden they manage.
-
get_short_name
()¶ Return the short name for the user.
-
has_orders
()¶ Determine whether the user has any orders at all.
-
is_anything
()¶ GardenHub is only useful if the logged-in user can manage any garden or plot. If not, that is very sad. :(
-
is_garden_manager
()¶ A garden manager is someone who facilitates renting Plots of a Garden out to Gardeners. Any person who is set as Garden.manager on at least one Garden.
-
is_gardener
()¶ A gardener is someone who rents a garden Plot and grows food there. Gardeners are assigned to Plot.gardener on at least one Plot. GM’s of a garden with plots are also considered gardeners.
-
is_order_picker
(order)¶ Is the user assigned as a picker on this order? True if the user is listed in Order.plot.garden.pickers. False otherwise.
-
is_picker
()¶ A picker is someone who is assigned to fulfill Orders on a Garden. They will submit Picks over the duration of the Orders.
-
exception